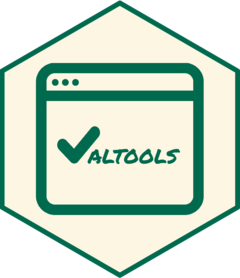
Manage dynamic refrerencing
vt_dynamic_referencer.Rd
Manage dynamic refrerencing
Manage dynamic refrerencing
Details
The job of the dynamic referencer is to aggregate the references that exist from requirements, test cases, test code and in the validation report and update them accordingly. This helps with the painful cases where a new reference may be added between existing numbering and all subsequent references need to be updated
The user should not need to use this object directly, it will be called upon on rendering of the contents in the validation report.
By default, the expected indicator for a reference is "##", though this can be changed. A reference starts with the indicator (##) and can then be any contiguous (no white spaces or special characters) alphanumeric sequence, or include an underscore or dash. ie. ##req:THISIS_A-reference1234 is valid for the entire string, but ##req:THISIS_A-reference.12345 is a dynamic reference up to the ".".
The method 'scrape_references' takes in a vector of strings, and an indicator whether the input file text is a requirement ('req'), test case or test code ('tc'). This allows numbering to increase independently for each. However, every reference must be unique. ie. ##reference whether it shows up in a test case or requirement will be the same reference.
The method 'reference_insertion' takes a vector of strings and replaces references with their numeric values.
Methods
Method scrape_references()
collect references from text.
Arguments
text
character vector to collect references from.
type
type of file being converted; a requirement ('req'), test case or test code ('tc')
Examples
ref <- vt_dynamic_referencer$new()
ref$list_references()
ref$scrape_references("##req:new_reference")
ref$list_references()
Method reference_insertion()
replace references in text with values
Examples
ref <- vt_dynamic_referencer$new()
ref$list_references()
ref$scrape_references("##new_reference")
ref$list_references()
ref$reference_insertion("This is my ##new_reference")
Method list_references()
list references available and their value
Examples
ref <- vt_dynamic_referencer$new()
ref$list_references()
ref$scrape_references("##new_reference")
ref$list_references()
Method new()
create a new dynamic reference object
Usage
vt_dynamic_referencer$new(
reference_indicator = "##",
type = c("number", "letter")
)
Examples
reference <- vt_dynamic_referencer$new()
reference
#> <vt_dynamic_referencer>
#> Public:
#> clone: function (deep = FALSE)
#> initialize: function (reference_indicator = "##", type = c("number", "letter"))
#> list_references: function ()
#> reference_insertion: function (text)
#> scrape_references: function (text)
#> Private:
#> add_reference: function (reference_id, type = c("req", "tc"))
#> advance_reference: function (type = c("req", "tc"))
#> ref_iter_number: list
#> reference_indicator: ##
#> reference_indicator_regex: \#\#
#> references: list
#> type: number
## ------------------------------------------------
## Method `vt_dynamic_referencer$scrape_references`
## ------------------------------------------------
ref <- vt_dynamic_referencer$new()
ref$list_references()
#> list()
ref$scrape_references("##req:new_reference")
ref$list_references()
#> $`req:new_reference`
#> [1] 1
#>
## ------------------------------------------------
## Method `vt_dynamic_referencer$reference_insertion`
## ------------------------------------------------
ref <- vt_dynamic_referencer$new()
ref$list_references()
#> list()
ref$scrape_references("##new_reference")
ref$list_references()
#> list()
ref$reference_insertion("This is my ##new_reference")
#> [1] "This is my ##new_reference"
## ------------------------------------------------
## Method `vt_dynamic_referencer$list_references`
## ------------------------------------------------
ref <- vt_dynamic_referencer$new()
ref$list_references()
#> list()
ref$scrape_references("##new_reference")
ref$list_references()
#> list()