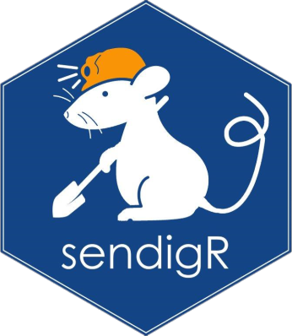
Extract the set of animals of the specified route of administration - or just add actual route of administration for each animal.
Source:R/getSubjRoute.R
getSubjRoute.Rd
Returns a data table with the set of animals included in the
animalList
matching the route of administration specified in the
routeFilter
.
If the routeFilter
is empty (null, na or empty string) - all rows from
animalList
are returned with an additional populated ROUTE column.
Usage
getSubjRoute(
dbToken,
animalList,
routeFilter = NULL,
exclusively = FALSE,
matchAll = FALSE,
inclUncertain = FALSE,
noFilterReportUncertain = TRUE
)
Arguments
- dbToken
Mandatory
Token for the open database connection (seeinitEnvironment
).- animalList
Mandatory, data.table.
A table with the list of animals to process.
The table must include at least columns named 'STUDYID' and 'USUBJID'.- routeFilter
Optional, character.
The route of administration value(s) to use as criterion for filtering of the input data table.
It can be a single string, a vector or a list of multiple strings.- exclusively
Mandatory if
routeFilter
is non empty, boolean.TRUE: Include animals only for studies with no other routes then included in
routeFilter
.FALSE: Include animals for all studies with route matching
routeFilter
.
- matchAll
Mandatory if
routeFilter
is non empty, boolean.TRUE: Include animals only for studies with route(s) matching all values in
routeFilter
.FALSE: Include animals for all studies with route matching at least one value in
routeFilter
.
- inclUncertain
Mandatory if
routeFilter
is non empty, boolean,.
Indicates whether animals for which the route cannot be confidently identified shall be included or not in the output data table.- noFilterReportUncertain
Mandatory if
routeFilter
is empty, boolean
Only relevant if therouteFilter
is empty.
Indicates if the reason should be included if the route cannot be confidently decided for an animal.
Value
The function returns a data.table with columns:
STUDYID (character)
Additional columns contained in the
animalList
tableROUTE (character)
The value is always returned in uppercase and trimmed for leading/trailing blanks.UNCERTAIN_MSG (character)
Included when parameterinclUncertain=TRUE
.
In case the ROUTE cannot be confidently matched during the filtering of data, the column contains an indication of the reason.
Is NA for rows where ROUTE can be confidently matched.
A non-empty UNCERTAIN_MSG value generated by this function is merged with non-empty UNCERTAIN_MSG values which may exist in the input set of animals specified inanimalList
- separated by '|'.NOT_VALID_MSG (character)
Included when parameternoFilterReportUncertain=TRUE
.
In case the ROUTE cannot be confidently decided, the column contains an indication of the reason.
Is NA for rows where the ROUTE can be confidently decided.
A non-empty NOT_VALID_MSG value generated by this function is merged with non-empty NOT_VALID_MSG values which may exist in the input set of animalsanimalList
- separated by '|'.
Details
The route of administration per animal are identified by a hierarchical lookup in these domains
EX - If a distinct not empty EXROUTE value is found for animal, this is included in the output.
TS - if a distinct TS parameter 'ROUTE' value exists for the study, this is included in the output.
The comparison of route values is done case insensitive and trimmed for leading/trailing blanks.
If input parameter inclUncertain=TRUE
, uncertain animals are included
in the output set. These uncertain situations are identified and reported (in
column UNCERTAIN_MSG):
TS parameter ROUTE is missing for study and no EX rows contain a EXROUTE value for the animal
The selected EXROUTE or TS parameter ROUTE value is invalid (not CT value - CDISC SEND code list ROUTE)
Multiple EXROUTE values have been found for the animal
Multiple TS parameter ROUTE values are registered for study but no EX rows contain a EXROUTE value for the animal
The found EXROUTE value for animal is not included in the TS parameter ROUTE value(s) registered for study
The same checks are performed and reported in column NOT_VALID_MSG if
routeFilter
is empty and noFilterReportUncertain=TRUE
.
Examples
if (FALSE) { # \dontrun{
# Extract animals administered oral or oral gavage plus uncertain animals
getSubjRoute(dbToken, controlAnimals,
routeFilter = c('ORAL', 'ORAL GAVAGE'),
inclUncertain = TRUE)
# Extract animals administered oral or oral gavage.
# Do only include studies which include both route values
getSubjRoute(dbToken, controlAnimals,
routeFilter = c('ORAL', 'ORAL GAVAGE'),
matchAll = TRUE)
# Extract animals administered subcutaneous.
# Include only animals from studies which do not contain other route values
getSubjRoute(dbToken, controlAnimals,
routeFilter = 'subcutaneous',
exclusively = TRUE)
# No filtering, just add ROUTE - do not include messages when
# these values cannot be confidently found
getSubjRoute(dbToken, controlAnimals,
noFilterReportUncertain = FALSE)
} # }